You can send data to a Raspberry Pi from an Arduino, have the Pi compute the data and use “intelligence” to send the Arduino a command. This allows you to fully use a Pi as a “Compute Module” for the Arduino with the ability to access databases, data-stores, API’s and anything else a “real” computer can do.
Prerequisites:
- Analog Temperature Sensor with Arduino
- Raspberry Pi – How to Begin Coding Python on Raspberry Pi
- Arduino – Send Commands with Serial Communication
- Arduino – Send Serial Commands from Raspbery Pi
- Arduino – Read Serial Communication with Raspberry Pi
Functional Parts in the Project:
- Arduino Uno – https://store.arduino.cc/usa/arduino-uno-rev3
- 560 Piece Jumper Wire Kit – https://amzn.to/2MsCLjL
- 220 Ohm Resistors – https://amzn.to/2RiiMD9
- Breadboard Kit – https://amzn.to/2Xih5ei
- LED Kit – https://amzn.to/2Rjhs2N
- Analog Temperature Sensor – https://amzn.to/2Rkkl3k
- Raspberry Pi
- USB Cable
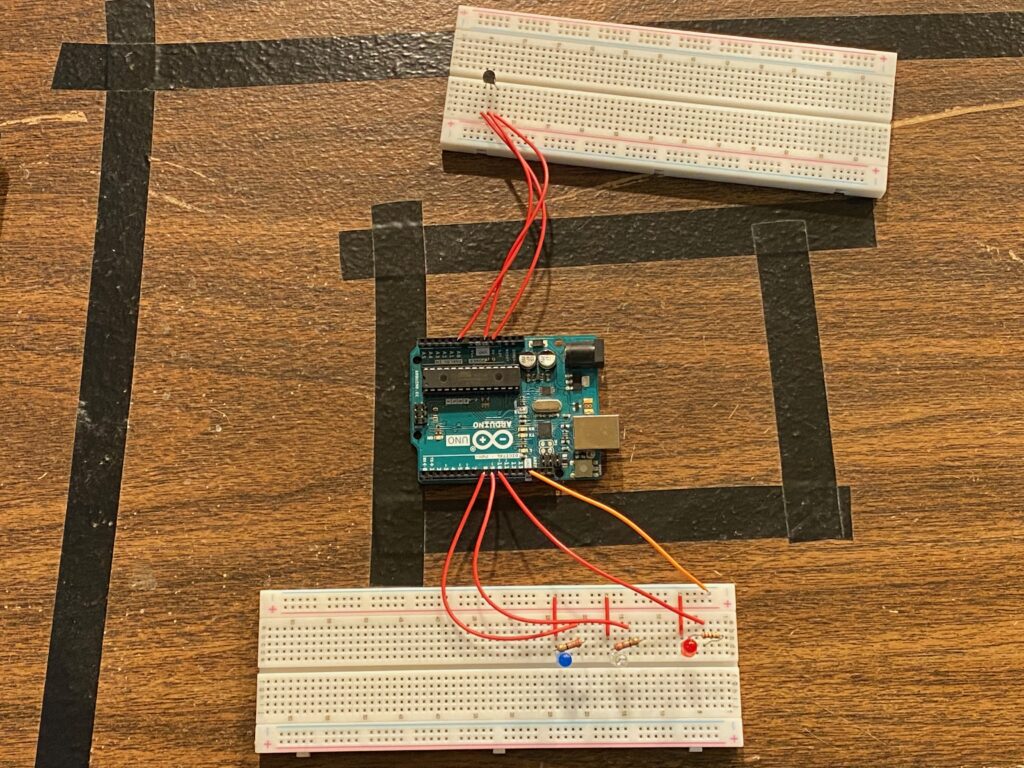

Arduino Sketch
String command;
#define sensorPin A0
#define blueLed 8
#define whiteLed 9
#define redLed 10
void setup() {
Serial.begin(9600);
pinMode(blueLed, OUTPUT);
pinMode(whiteLed, OUTPUT);
pinMode(redLed, OUTPUT);
}
void loop() {
int reading = analogRead(sensorPin);
float voltage = reading * 5.0;
voltage /= 1024.0;
float temperatureC = (voltage - 0.5) * 100 ;
float temperatureF = (temperatureC * 9.0 / 5.0) + 32.0;
Serial.println(temperatureF);
if (Serial.available()) {
command = Serial.readStringUntil('\n');
command.trim();
if (command.equals("white")) {
digitalWrite(whiteLed, HIGH);
digitalWrite(blueLed, LOW);
digitalWrite(redLed, LOW);
}
else if (command.equals("blue")) {
digitalWrite(whiteLed, LOW);
digitalWrite(blueLed, HIGH);
digitalWrite(redLed, LOW);
}
else if (command.equals("red")) {
digitalWrite(whiteLed, LOW);
digitalWrite(blueLed, LOW);
digitalWrite(redLed, HIGH);
}
else if (command.equals("all")) {
digitalWrite(whiteLed, HIGH);
digitalWrite(blueLed, HIGH);
digitalWrite(redLed, HIGH);
}
else if (command.equals("off")) {
digitalWrite(whiteLed, LOW);
digitalWrite(blueLed, LOW);
digitalWrite(redLed, LOW);
}
else {
digitalWrite(whiteLed, HIGH);
digitalWrite(blueLed, HIGH);
digitalWrite(redLed, HIGH);
}
}
delay(1000);
}
Raspberry Python Script
- Remember that ttyACM0 may iterate up to ttyACM1
import serial
if __name__ == '__main__':
ser = serial.Serial('/dev/ttyACM0',9600, timeout=1)
ser.flush()
while True:
if ser.in_waiting > 0:
line = ser.readline().decode('utf-8').rstrip()
print(line)
if float(line) <= 60:
ser.write(b"blue\n")
elif float(line) > 60 and float(line) <= 70:
ser.write(b"white\n")
elif float(line) > 70:
ser.write(b"red\n")
else:
ser.write(b"all\n")
Be the first to comment