This project allows you to get a temperature value from the Arduino, pass it to the Raspberry Pi, have a Python Script process that value and store it in a file, then have a PHP Script read the file and create a dynamic web page. This is a simple project, but shows the concept of designing a system that hands tasks between different physical devices and programming languages.
Prerequisites:
- Analog Temperature Sensor with Arduino
- Raspberry Pi – How to Begin Coding Python on Raspberry Pi
- Arduino – Send Commands with Serial Communication
- Arduino – Send Serial Commands from Raspbery Pi
- Arduino – Read Serial Communication with Raspberry Pi
- PHP Programming (2019)
Functional Parts in the Project:
- Arduino Uno – https://store.arduino.cc/usa/arduino-uno-rev3
- 560 Piece Jumper Wire Kit – https://amzn.to/2MsCLjL
- Breadboard Kit – https://amzn.to/2Xih5ei
- Analog Temperature Sensor – https://amzn.to/2Rkkl3k
- Raspberry Pi
- USB Cable
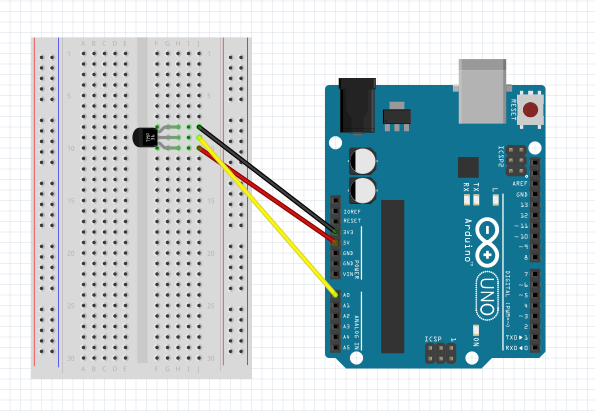
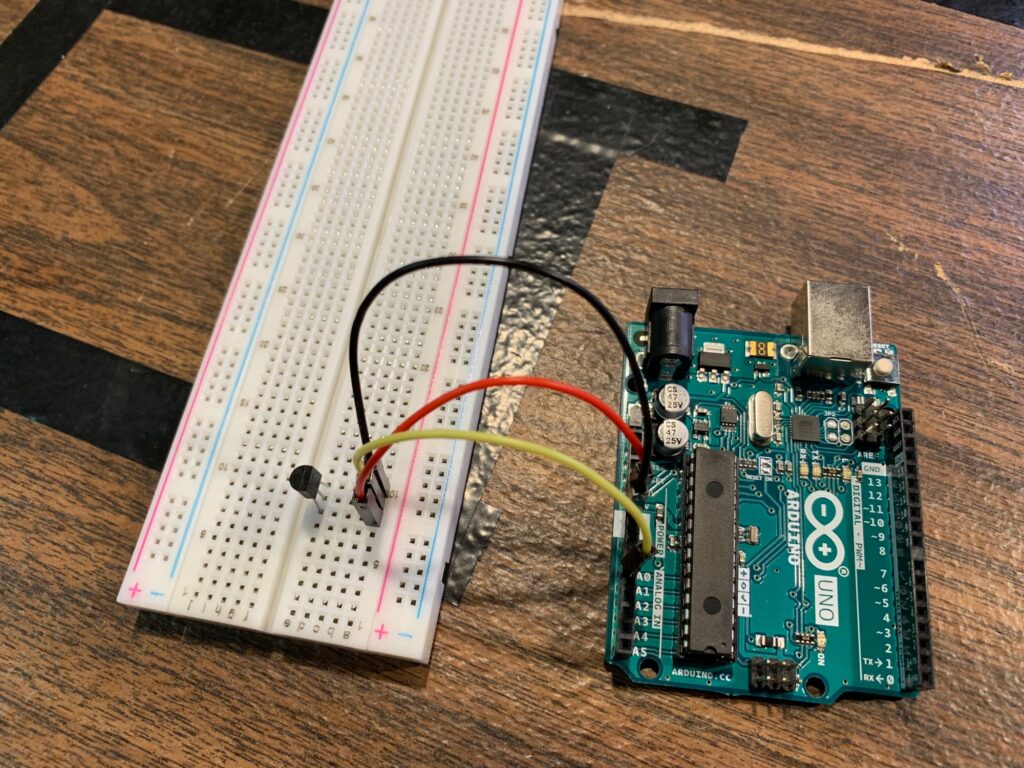
Arduino Sketch
#define sensorPin A0
void setup() {
Serial.begin(9600);
}
void loop() {
int reading = analogRead(sensorPin);
float voltage = reading * 5.0;
voltage /= 1024.0;
float temperatureC = (voltage - 0.5) * 100 ;
float temperatureF = (temperatureC * 9.0 / 5.0) + 32.0;
Serial.println(temperatureF);
delay(1000);
}
Install Apache on Raspberry Pi
sudo apt-get install apache 2
Install PHP on Raspberry Pi
sudo apt-get install php
Change Ownership of HTML Folder on Raspberry Pi
sudo chown pi /var/www/html
Python Script
import serial
if __name__ == '__main__':
ser = serial.Serial('/dev/ttyACM1',9600, timeout=1)
ser.flush()
while True:
if ser.in_waiting > 0:
line = ser.readline().decode('utf-8').rstrip()
file = open("/var/www/html/data.txt","w")
file.write(line)
file.close()
print(line)
tempApp.php PHP Script
<meta http-equiv="refresh" content="5">
<?php
$temp = file_get_contents("data.txt");
if ($temp >= 70){
$color="red";
}else if($temp >= 60 && $temp < 70){
$color="green";
} else if($temp < 60){
$color="blue";
} else {
$color="black";
}
print "<h1 style='text-align:center;'>Arduino/Raspberry Pi Temp Web Device</h1>";
print "<br>";
print "<p style='text-align:center; font-size:200px; margin-top:10px; margin-bottom:10px; color:".$color.";'>".$temp."</p>";
?>
Get IP Address of Raspberry Pi
sudo ifconfig
sudo ip address
On another computer go to the xxx.xxx.xxx.xxx/tempApp.php in the web browser

Be the first to comment