In this project we are going to use an Arduino to get the room temperature, and be able to turn on a regular house fan. The Arduino sends the temperature value to the Raspberry Pi using the Serial Communication, the Pi’s Python script takes the value and saves it to a text file. A PHP script then dynamically builds a web page and includes a link to turn the fan on or off. When clicked this changes the value in a text file, the python script reads the text file, and sends a command to the Arduino.
Prerequisites
- Arduino – Send Commands with Serial Communication
- Arduino – Read Serial Communication with Raspberry Pi
- Arduino – Send Serial Commands from Raspbery Pi
- Arduino – Bidirectional Serial Communication with Raspberry Pi
- Arduino – Temperature Web App with Raspberry Pi
Functional Parts in the Project:
- Arduino Uno – https://store.arduino.cc/usa/arduino-uno-rev3
- 560 Piece Jumper Wire Kit – https://amzn.to/2MsCLjL
- Breadboard Kit – https://amzn.to/2Xih5ei
- Analog Temperature Sensor – https://amzn.to/2Rkkl3k
- Raspberry Pi
- USB Cable
- DLI IoT Relay – https://amzn.to/2zPR3ou
- Fan or Other Plugin electric Device
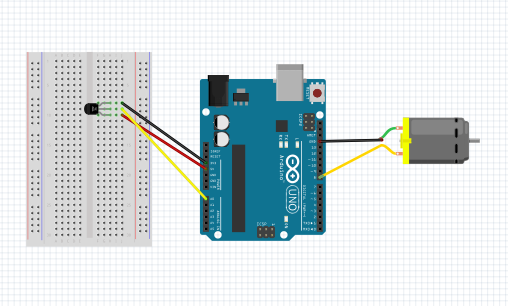
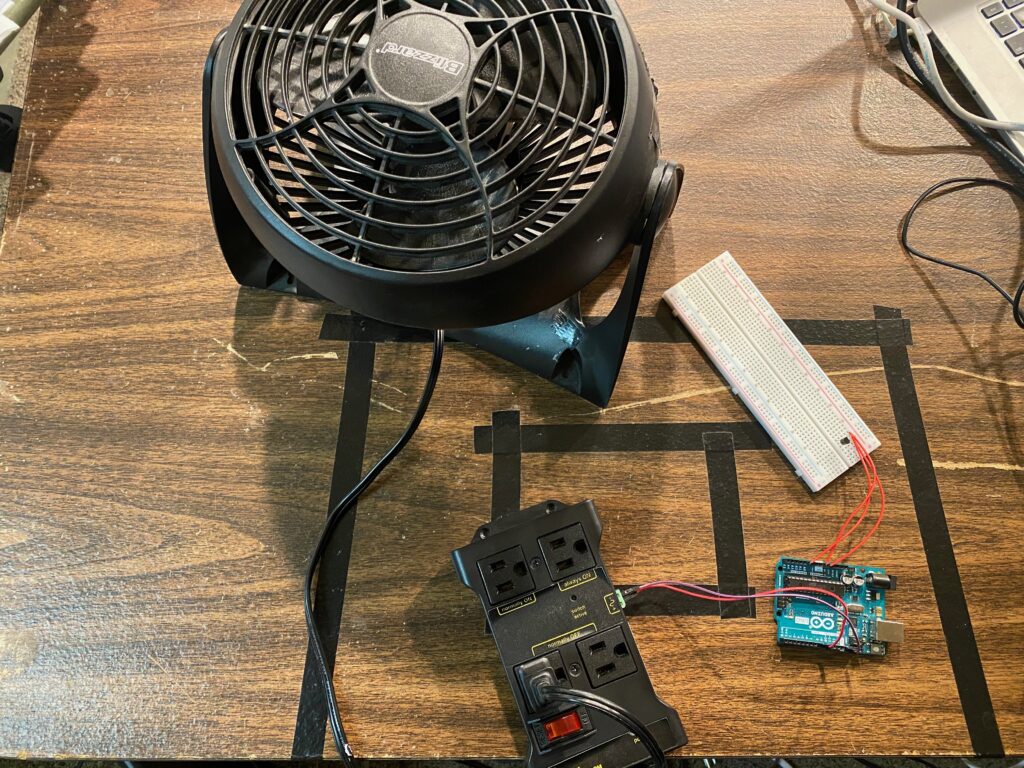
Arduino Sketch
String command;
#define sensorPin A0
#define fan 8
void setup() {
Serial.begin(9600);
pinMode(fan, OUTPUT);
}
void loop() {
int reading = analogRead(sensorPin);
float voltage = reading * 5.0;
voltage /= 1024.0;
float temperatureC = (voltage - 0.5) * 100 ;
float temperatureF = (temperatureC * 9.0 / 5.0) + 32.0;
Serial.println(temperatureF);
if (Serial.available()) {
command = Serial.readStringUntil('\n');
command.trim();
if (command.equals("on")) {
digitalWrite(fan, HIGH);
}
else if (command.equals("off")) {
digitalWrite(fan, LOW);
}
else {
digitalWrite(fan, HIGH);
delay(500);
digitalWrite(fan, LOW);
}
}
delay(1000);
}
Linux Setup
sudo apt-get install apache2
sudo apt-get install php
sudo chown pi /var/www/html
sudo chmod 777 /var/www/html/data.txt
sudo chmod 777 /var/www/html/fanStatus.txt
fanApp.py
import serial
if __name__ == '__main__':
ser = serial.Serial('/dev/ttyACM0',9600, timeout=1)
ser.flush()
while True:
if ser.in_waiting > 0:
line = ser.readline().decode('utf-8').rstrip()
tempFile = open("/var/www/html/data.txt","w")
tempFile.write(line)
tempFile.close()
print("***")
fanFile = open("/var/www/html/fanStatus.txt","r")
command = fanFile.read()
fanFile.close()
print(line)
if command == 'on':
ser.write(b"on\n")
print(command)
elif command == 'off':
ser.write(b"off\n")
print(command)
else:
print("error")
fanApp.php
<meta http-equiv="refresh" content="5">
<?php
print "<h1 style='text-align:center;'>Arduino/Raspberry Pi Temp Web Device</h1>";
print "<br>";
$temp = file_get_contents("data.txt");
$fanSwitch;
$fanTrigger = $_GET["fanSwitch"];
if ($fanTrigger == "on" || $fanTrigger == "off"){
file_put_contents("fanStatus.txt", $fanTrigger);
}
$fanStatus = file_get_contents("fanStatus.txt");
if ($temp > 70){
$color="red";
}else {
$color="green";
}
if ($fanStatus == "on"){
$fanSwitch = "off";
} else if ($fanStatus == "off"){
$fanSwitch = "on";
} else {
$fanSwitch = "problem";
}
print "<p style='text-align:center; font-size:200px; margin-top: 10px; margin-bottom: 10px;color:".$color.";'>".$temp."</p>";
print "<p>Fan is ".$fanStatus."</p>";
print "<a href='fanApp.php?fanSwitch=".$fanSwitch."'>Turn Fan ".$fanSwitch."</p></a>";
?>
Be the first to comment