- 00:40 Intro
- 00:51 What is DALL E API
- 09:00 Demo of DALL E API
- 14:25 Getting OpenAI API Key
- 21:54 Create Images with DALL E
- 30:17 Download DALL E Images
- 35:04 Edit Images with DALL E
- 39:10 Create Image variations with DALL E
- 44:53 Final Thoughts
Setup:
Get API Key from OpenAI.com
pip3 install openai
Create Images with DALL E:
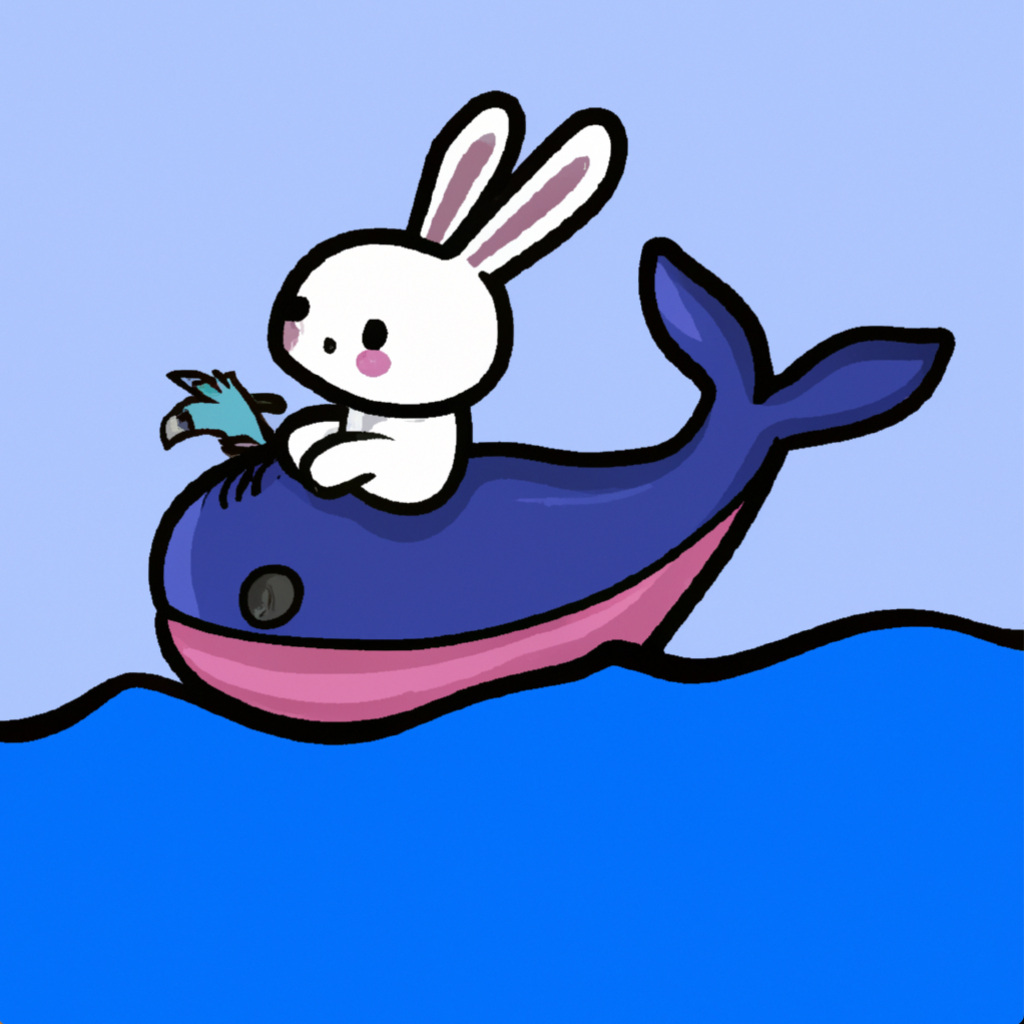
openai.api_key = 'APIKEY'
response = openai.Image.create(
prompt="A kid riding a cat photo realistic",
n=3,
size="1024x1024"
)
print("JSON Response")
print(response)
print("First/ Only URL Result")
print(response["data"][0]["url"])
print("All URLs")
for x in response["data"]:
print(x["url"])
print("****")
Download DALL E Images:
import openai
from time import time, sleep
import urllib.request
openai.api_key = 'APIKEY'
response = openai.Image.create(
prompt="A cartoon bunny on a whale",
n=4,
size="1024x1024"
)
print("All URLs")
for x in response["data"]:
name = time()
name = f'{int(name)}.png'
urllib.request.urlretrieve(x["url"], name)
print(x["url"])
print(name)
print("****")
sleep(1.5)
Edit Image with DALL E:

import openai
openai.api_key = 'APIKEY'
response = openai.Image.create_edit(
image=open("musk.png", "rb"),
prompt="at a hot dog stand on mars",
n=1,
size="1024x1024"
)
for x in response["data"]:
print(x["url"])
print("")
Create Variation of Image with DALLE:

import openai
openai.api_key = 'APIKEY'
image = "pets.png"
response = openai.Image.create_variation(
image=open(image, "rb"),
n=5,
size="1024x1024"
)
print(response)
file = open("variation.html", "w")
file.write(f'<img style="height:250;width:250;border:3px solid green;" src="{image}">')
for x in response["data"]:
file.write(f'<img style="height:250;width:250;border:3px solid red;" src="{x["url"]}">')
print(x["url"])
file.close()
Be the first to comment