I2C devices allow you to add numerous sensors and displays to your Arduino project using only 2 communication wires. The issue can be that since I2C is an Addressable Communication Protocol that you need to know the addresses of the I2C devices. This scanner sketch will allow you to determine what the addresses are of your I2C devices.
Functional Parts in the Project:
- Arduino Uno – https://store.arduino.cc/usa/arduino-uno-rev3
- 20 x 4 I2C LCD Screen – https://amzn.to/2JVuKzn
- 560 Piece Jumper Wire Kit – https://amzn.to/2MsCLjL
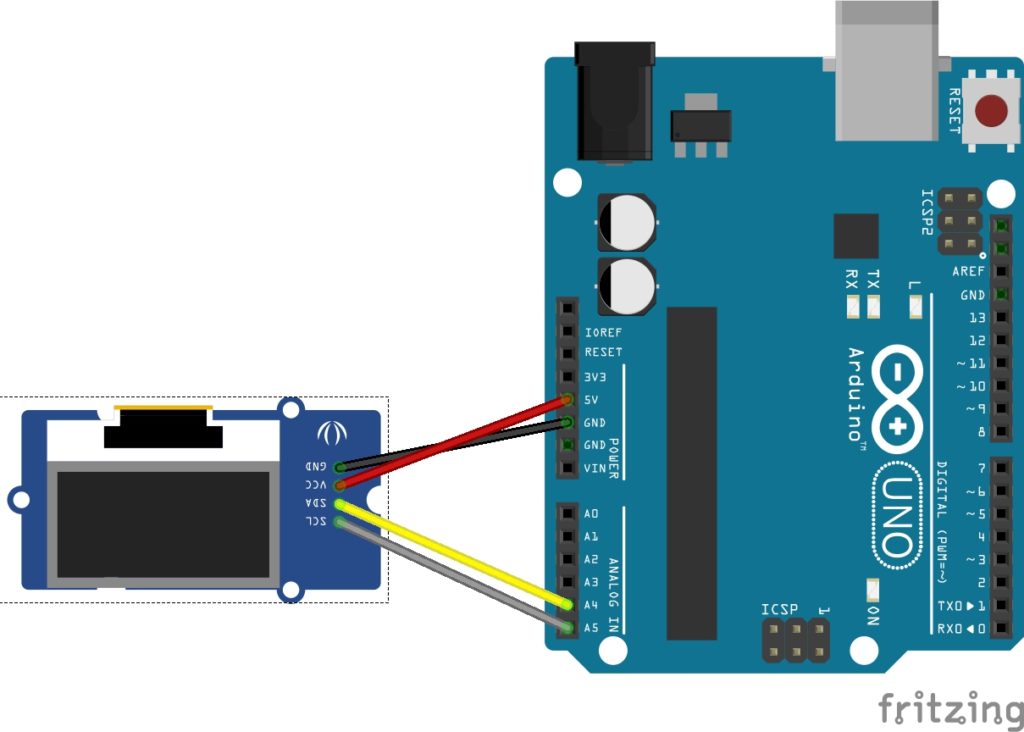

#include <Wire.h>
void setup()
{
Wire.begin();
Serial.begin(9600);
}
void loop()
{
int error;
int address;
int devices = 0;
Serial.println("Devices found:");
for(address = 1; address < 127; address++ )
{
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0)
{
Serial.print("0x");
if (address<16)
Serial.print("0");
Serial.println(address,HEX);
devices++;
}
else if (error==4)
{
Serial.print("Unknown error at address 0x");
if (address<16)
Serial.print("0");
Serial.println(address,HEX);
}
}
if (devices == 0)
Serial.println("No I2C devices found");
delay(5000);
}
Be the first to comment