You can use an Arduino Uno with WiFi to constantly check on the ping response from servers and networked devices.
Libraries Required for this Demonstration:
- Adafruit SSD1306
- Adafruit GFX
Note: Remember to change the I2C address within the example sketch.
Functional Parts in the Project:
- Arduino WiFi Rev 2 – https://store.arduino.cc/usa/arduino-uno-wifi-rev2
- 128 x 64 OLED – https://amzn.to/2Mc8Fz2
- Breadboard Kit – https://amzn.to/2Xih5ei
- 560 Piece Jumper Wire Kit – https://amzn.to/2MsCLjL
- LED Kit – https://amzn.to/2Rjhs2N
- 220 Ohm Resistors – https://amzn.to/2RiiMD9
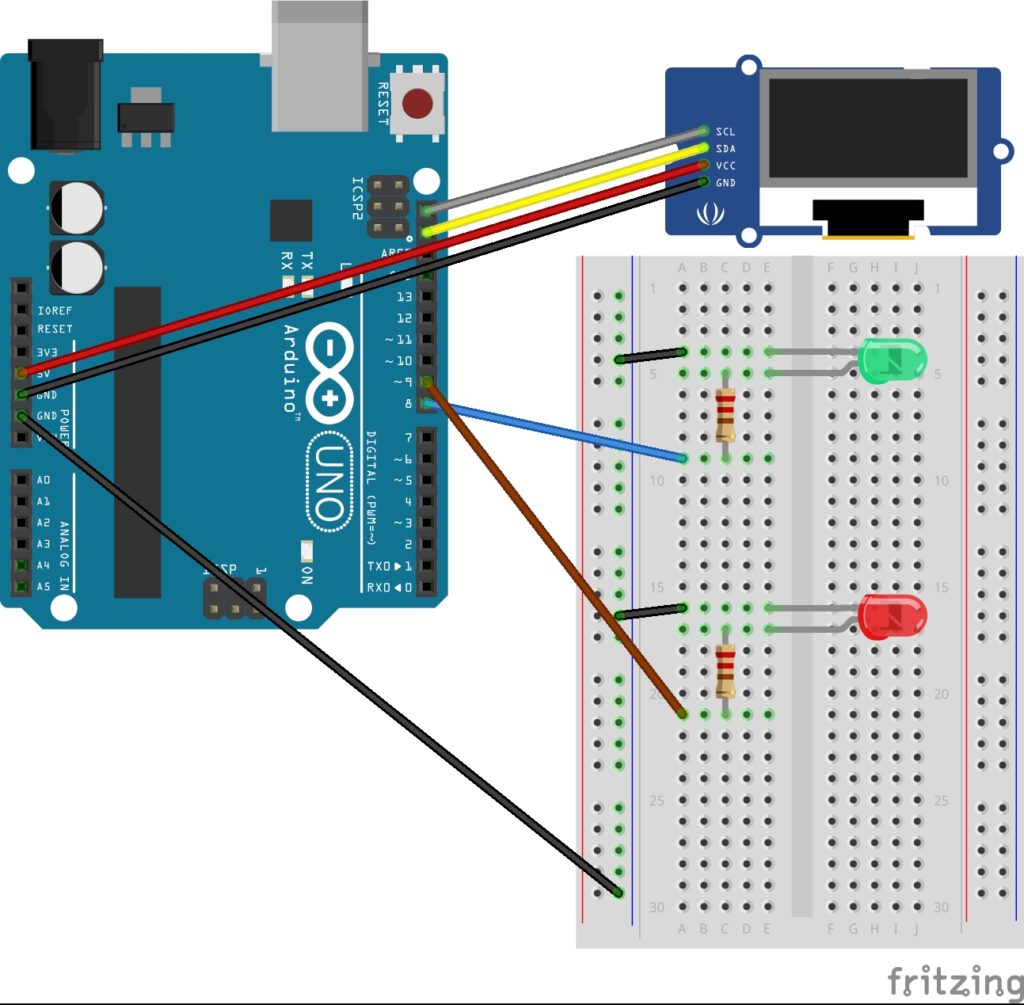
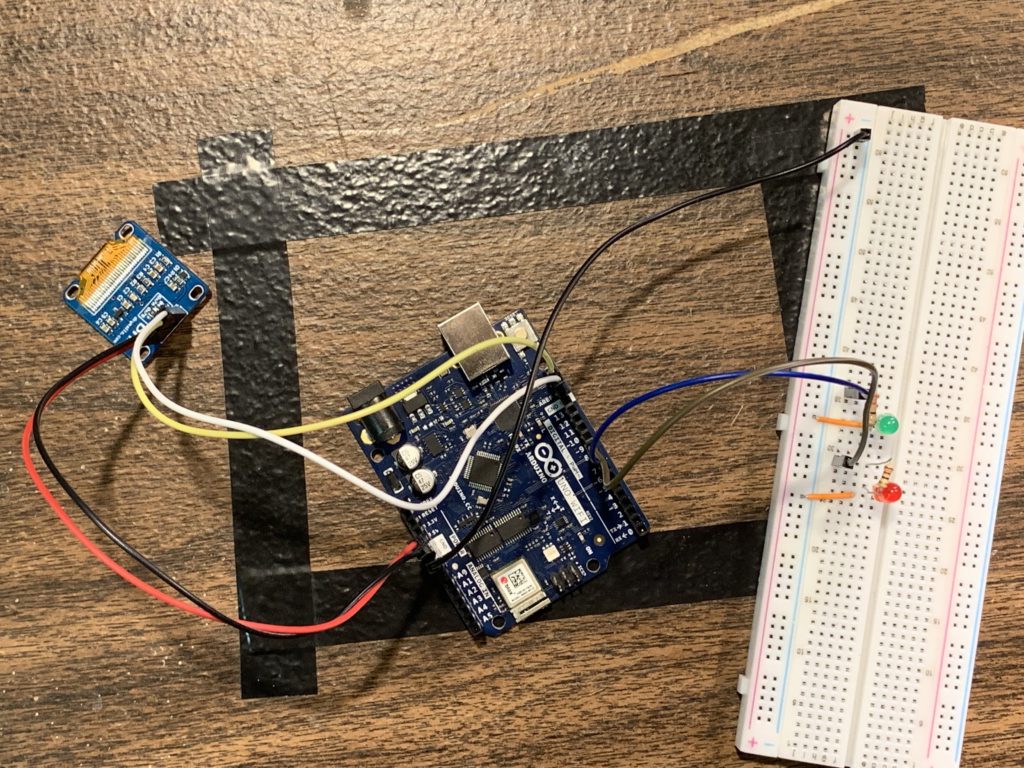
#include <Adafruit_SSD1306.h>
#include <Adafruit_GFX.h>
#include <WiFiNINA.h>
#define greenLED 8
#define redLED 9
#define OLED_WIDTH 128
#define OLED_HEIGHT 64
#define OLED_ADDR 0x3C
Adafruit_SSD1306 display(OLED_WIDTH, OLED_HEIGHT);
char ssid[] = "test";
char pass[] = "";
int status = WL_IDLE_STATUS;
String google = "www.google.com";
String salesforce = "www.salesforce.com";
IPAddress localServer(172, 16, 42, 5);
int googleResult;
int salesforceResult;
int serverResult;
int gatewayResult;
void setup() {
pinMode(greenLED, OUTPUT);
pinMode(redLED, OUTPUT);
Serial.begin(9600);
display.begin(SSD1306_SWITCHCAPVCC, OLED_ADDR);
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to Network named: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
delay(10000);
}
String ssid = WiFi.SSID();
IPAddress ip = WiFi.localIP();
IPAddress gateway = WiFi.gatewayIP();
IPAddress subnet = WiFi.subnetMask();
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(WHITE);
display.setCursor(0, 0);
display.println("Net Tester");
display.setTextSize(1);
display.setTextColor(WHITE);
display.setCursor(0, 17);
display.print("SSID: ");
display.println(ssid);
display.print("IP: ");
display.println(ip);
display.print("Subnet: ");
display.println(subnet);
display.print("Gateway:");
display.println(gateway);
display.display();
delay(5000);
}
void loop() {
IPAddress gateway = WiFi.gatewayIP();
googleResult = WiFi.ping(google);
salesforceResult = WiFi.ping(salesforce);
serverResult = WiFi.ping(localServer);
gatewayResult = WiFi.ping(gateway);
long counter = millis();
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(WHITE);
display.setCursor(0, 0);
display.println("Net Tester");
display.setTextSize(1);
display.setTextColor(WHITE);
display.setCursor(0, 17);
display.print("Gateway: ");
display.println(gatewayResult);
display.print("Local Server: ");
display.println(serverResult);
display.print("Google: ");
display.println(googleResult);
display.print("Salesforce: ");
display.println(salesforceResult);
display.println(counter);
display.display();
if (gatewayResult < 0 || serverResult < 0 || googleResult < 0 || salesforceResult < 0) {
digitalWrite(greenLED, LOW);
digitalWrite(redLED, HIGH);
} else {
digitalWrite(greenLED, HIGH);
digitalWrite(redLED, LOW);
}
delay(5000);
}
Be the first to comment